An application serves as a gateway for customers to interact with your products and services from anywhere with an internet connection. Consider your application like a secure locker where valuable data and sensitive information is stored. Now, imagine a hacker getting in but not with a big hammer, but by using tricky lines of malicious code.
When a hacker breaks in, it is not a small problem or just a computer issue, it can be a big hit for your organization’s reputation and it can take a very long time to fix everything.
In this tech-driven world, ensuring the security of applications is paramount. It not only prepares you for current or future cyber threats but helps you identify and mitigate potential security risks before attackers do.
In 2018, Marriott International experienced a massive data breach where hackers gained unauthorized access to the reservation system of its subsidiary, Starwood. The breach impacted approximately 500 million guests and exposed their personal details, passport numbers, and payment information. This case emphasized the importance of robust application security.
Understanding Application Security
At its core, application security, often abbreviated as AppSec, is the practice of protecting software applications from various threats and vulnerabilities throughout their development, deployment, and maintenance lifecycles. It encompasses a holistic approach designed to safeguard an application’s integrity, confidentiality, and availability.
Application security aims to mitigate risk associated with unauthorized access, data breaches, and other security incidents protecting software application code and data against cyber threats.
Within the realm of application security, specific focus areas include:
- Web application security:
Web applications that run on web servers and are accessed via an internet connection are inherently vulnerable because they accept connections over insecure networks. Because of the critical nature of many web applications that house sensitive customer data, they become lucrative targets for attackers, emphasizing the importance of strong cybersecurity measures.
- Mobile application security:
Mobile application security is concerned with ensuring the safety of mobile applications, addressing challenges specific to mobile platforms, and safeguarding sensitive data stored on or transmitted from these devices.
- API security:
Application Programming Interface (API) security entails securing the interfaces through which applications communicate and share data, as well as preventing unauthorized access and data breaches via API endpoints.
- Secure Code Review:
Secure code review is a critical aspect of software development, aimed at ensuring the security and integrity of the code before it’s deployed. This process involves a detailed examination of the source code to identify any security vulnerabilities, coding errors, or non-compliance with best coding practices.
Tailored application defenses
Organizations deploy a variety of tools, programs, services and devices as guardians against unauthorized access. Firewalls, antivirus systems, and data encryption stand among the frontline defenses, creating barriers to prevent unauthorized users from breaching the system. However, for organizations aiming to safeguard specific, sensitive data sets, the establishment of unique application security policies becomes imperative.
While the journey of application security unfolds in stages, the most critical juncture for establishing best practices is frequently during the development phases. Here are several practices that organizations can incorporate, either during the development or post-development phase, to enhance the security posture of their applications:
- Static analysis and code reviews:
Review and analyze code on a regular basis for potential vulnerabilities and security flaws. Static analysis tools can aid in the detection of problems before the application is deployed.
- Developer security training:
Provide developers with ongoing security training to ensure they are aware of the most recent security best practices and can incorporate them into their coding habits.
- Penetration testing:
Conduct penetration testing to simulate real-world attacks and identify potential security flaws in the application.
- Dependency scanning:
Scan and update third-party libraries and dependencies on a regular basis to patch known vulnerabilities and ensure the overall security of the application.
- Authentication and authorization controls:
Implement strong authentication mechanisms and precise authorization controls to ensure that only authorized users have access and prevent unauthorized entry.
Common Application Security threats you should know about
There are numerous security threats that affect software applications. Nonetheless, the Top 10 list of the Open Web Application Security Project (OWASP) aggregates the most common and severe application threats to impact production applications.
- Injection
When untrusted data is sent to an interpreter as part of a command or query, an injection attack occurs. This could result in unauthorized access, data manipulation, or even system compromise. SQL injection is the most common, but it can also affect NoSQL, operating systems, and LDAP servers.
Example Vulnerable Code
from flask import Flask, request |
In this snippet, the application is fetching a user’s details from a database based on a username provided in the URL’s query parameters. However, the code directly includes the username parameter in the SQL query without any sanitization or validation, making it vulnerable to SQL injection.
Exploitation using an HTTP Request
An attacker could exploit this vulnerability by sending a specially crafted HTTP request. For example:
http://example.com/user?username=' OR '1'='1 |
This request would change the SQL query to:
SELECT * FROM users WHERE username = '' OR '1'='1' |
This modified query will always evaluate as true, potentially returning sensitive information about all users in the database.
Way to Fix It
To prevent this kind of attack, you should never directly include user input in SQL queries. Instead, use parameterized queries or prepared statements. Here’s the revised code:
@app.route('/user') |
In this fixed version, the ? is a placeholder that gets replaced with the username variable in a way that’s safe from SQL injection. The database engine properly escapes the user input, ensuring that it is treated as data and not as part of the SQL command.
- Broken Authentication
Many applications’ authentication and authorization functions are insufficient or broken. This makes it possible for an attacker to steal user credentials or gain access without them.By implementing secure session management and authentication and verification for all identities, identity attacks and exploits can be avoided.
Vulnerable Code Snippet:
A web application that handles user sessions insecurely.
@app.route('/login', methods=['POST']) |
In this example, the application does not implement any mechanisms to prevent session fixation attacks, and it lacks multifactor authentication, making it vulnerable to various forms of authentication attacks.
Exploitation:
An attacker could exploit this by hijacking a user session or using session fixation techniques.
Fix:
To address these vulnerabilities, you can implement the following:
- Strong Session Management: Use secure, unique session tokens that are regenerated after login. Also, implement session expiration and timeouts.
- Multi-Factor Authentication (MFA): Add an additional layer of security by requiring a second factor, such as a one-time password (OTP) or a biometric factor, in addition to the regular username and password.
- Account Lockout Mechanisms: Implement account lockouts after a certain number of failed login attempts to prevent brute-force attacks.
- Regularly Update Authentication Mechanisms: Stay updated with the latest security practices and patches for the authentication mechanisms in use.
- Cryptographic Failures
Cryptographic failures (also known as “sensitive data exposure”) involve the disclosure of sensitive data, such as credit-debit card numbers, other banking details, or personal information, as a result of insufficient encryption, insufficient storage practices, or ineffective data transmission protocols.
This application security risk may result in noncompliance with data privacy regulations such as GDPR and financial standards such as PCI DSS.
Example Code Snippet:
Storing user passwords in plain text.
# Flask [email protected]('/register', methods=['POST']) |
Exploitation:
If the database is compromised, attackers gain direct access to user passwords.
Fix:
Use a strong hashing algorithm like bcrypt for storing passwords.
- XML External Entities (XXE)
XXE attacks exploit vulnerable XML processors, allowing attackers to read internal files, scan internal networks, and execute remote code. Proper validation of XML inputs is crucial to prevent such attacks.
Vulnerable Code Snippet:
An application parses XML input without disabling external entities.
# Python example using XML |
Exploitation:
An attacker submits an XML document that references an external entity, like a file on the server.
Fix:
Disable external entities in the XML parser.
- Broken Access Control
Flaws in access controls can lead to unauthorized access to privileged functionalities or sensitive data. This includes improper user permissions, which may result in data breaches or unauthorized actions. In simple terms, it allows attackers to gain unauthorized access to user accounts and act as administrators or ordinary users.
Vulnerable Code Snippet:
No check for user roles in accessing admin functionality.
@app.route('/admin') |
Exploitation:
A regular user accesses /admin and reaches the admin panel.
Fix:
Implement role-based access control.
- Security Misconfigurations
Even if an application includes security features, they can be configured incorrectly. This is common because no one altered the application’s default configuration. Failure to patch operating systems and frameworks is one example.
Vulnerable Code Snippet:
A database connection with default credentials.
# Example of database |
Exploitation:
An attacker uses default credentials to gain access to the database.
Fix:
Change default credentials and configure database securely.
- Cross-Site Scripting (XSS)
XSS occurs when an application allows untrusted data to be included in a web page, leading to the execution of malicious scripts in the user’s browser. Attackers can use this to steal information or perform actions on behalf of the user.
Vulnerable Code Snippet:
Displaying user input directly in a web page.
@app.route('/search') |
Exploitation:
An attacker injects a script tag in the query parameter, which gets executed in the browser.
Fix:
Escape user input before displaying it on the page.
- Insecure Deserialization:
Insecure deserialization vulnerabilities can enable attackers to execute arbitrary code, compromise the application, or conduct further attacks. It occurs when untrusted data is used to instantiate objects during the deserialization process.
Vulnerable Code Snippet:
Deserializing user-provided data without validation.
import pickle |
Exploitation:
An attacker sends a maliciously crafted object, leading to code execution on the server.
Fix:
Avoid deserializing data from untrusted sources or use safe serialization formats like JSON.
- Vulnerable and Outdated Components
Vulnerable and outdated components (also known as “using components with known vulnerabilities”) include any vulnerability caused by outdated or unsupported software. It can happen if you build or use an application without first learning about its internal components and versions.
Vulnerable Code Snippet:
Using an outdated library with known vulnerabilities.
import some_old_library |
Exploitation:
Exploit known vulnerabilities in the outdated library.
Fix:
Regularly update libraries and dependencies to their latest, secure versions.
- Security Logging and Monitoring Failures
Failures in security logging and monitoring, previously referred to as “insufficient logging and monitoring,” are caused by ineffective practices that impede the timely detection of security incidents. Organizations risk being unaware of attacks if comprehensive logs and effective monitoring are not in place, resulting in a failure to recognize and respond to security threats, allowing them to linger undetected.
Vulnerable Code Snippet:
An application without proper logging for failed login attempts or system errors.
@app.route('/login', methods=['POST']) |
In this example, the application fails to log failed login attempts, which could be an indicator of a brute-force attack.
Exploitation:
An attacker may attempt multiple login attempts to guess user credentials. Without proper logging, these attempts can go unnoticed, increasing the risk of a successful attack.
Fix:
Implement comprehensive logging for all critical actions, including failed logins, system errors, and other anomalies. This should also include user and administrative actions within the application to detect any unauthorized or suspicious activities. Additionally, ensure proper monitoring and alert systems are in place to quickly respond to potential security incidents.
import logging |
Secure apps with Application Security Testing
What is Application Security testing?
Application security testing is the systematic evaluation of software applications to identify and rectify security flaws and vulnerabilities. The primary goal of this testing is to ensure that an application is resistant to potential security threats and attacks. A few techniques companies use include:
- White box security reviews
A security expert conducts a thorough examination of an application’s source code during a white-box security review. The expert meticulously walks through the codebase during this process, scrutinizing each line for potential security flaws and coding issues.
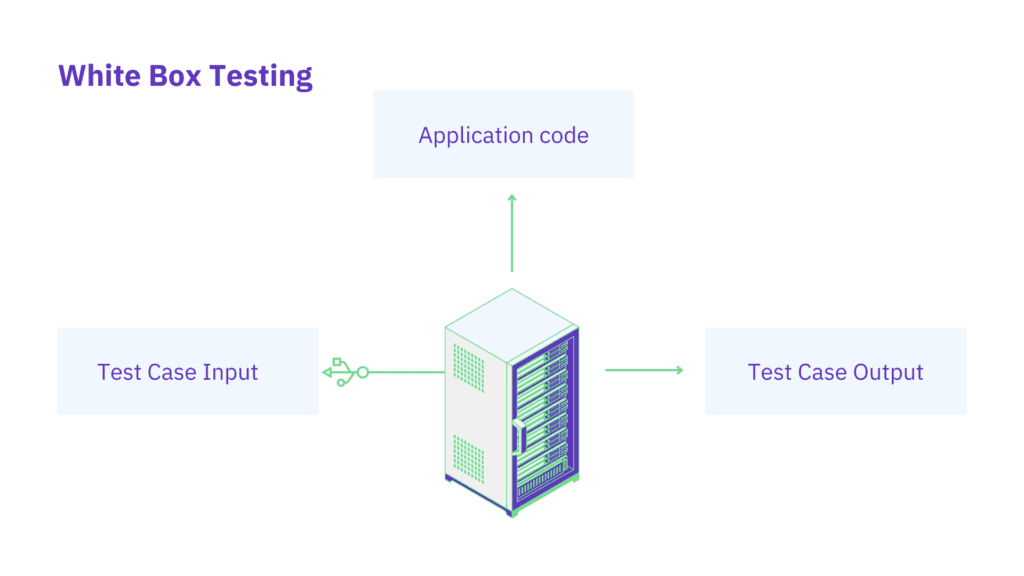
- Black box security reviews
Blackbox security reviews attempt to hack the application in order to simulate real-world attacks. Security experts, also known as ethical hackers, use a variety of techniques to probe the application’s defenses, simulating the actions of malicious actors.
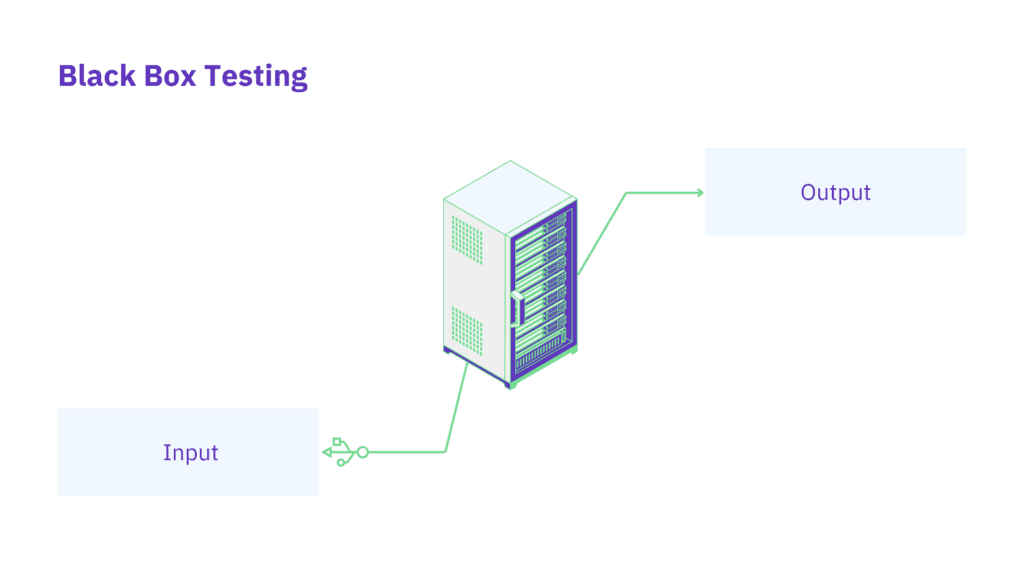
Application Security testing tools
- Static Application Security Testing (SAST)
White box testers use SAST tools to inspect the inner workings of applications. It involves inspecting static source code and reporting on security flaws discovered.
SAST can assist in locating issues in non-compiled code such as syntax errors, input validation issues, invalid or insecure references, or math errors. SAST can be applied to compiled code using binary and byte-code analyzers.
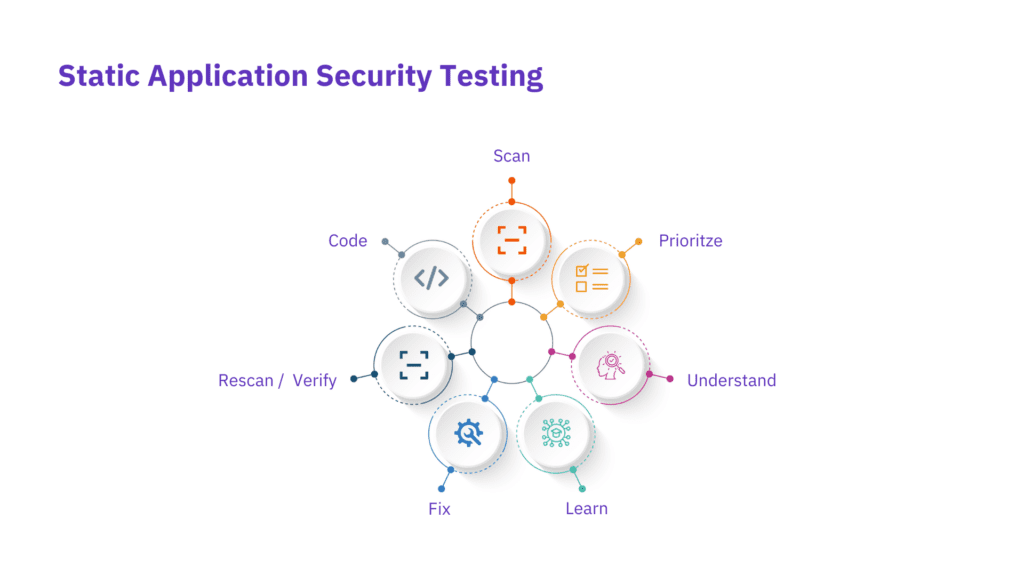
- Dynamic Application Security Testing (DAST)
DAST tools help black box testers execute and inspect code at runtime. It aids in the detection of issues that may represent security vulnerabilities. DAST is used by organizations to perform large-scale scans that simulate multiple malicious or unexpected test cases. These tests provide feedback on the application’s performance.
DAST can assist in identifying issues such as query strings, script usage, requests and responses, authentication, cookie and session handling, third-party component execution, DOM injection, and data injection.
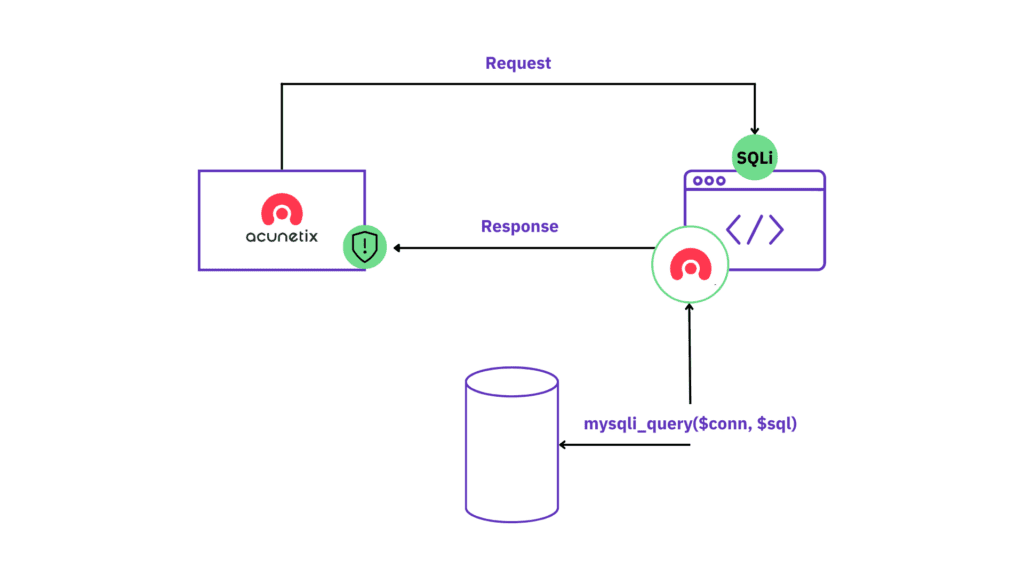
- Interactive Application Security Testing (IAST)
SAST and DAST are combined in IAST, making it a hybrid approach. The interactive approach to security testing combines static and dynamic analysis, allowing you to identify known vulnerabilities while also determining whether they are used in the running application and can be exploited.
IAST tools can simulate complex attack patterns and collect detailed information about application execution flow and data flows. It can check how an application responds while performing a dynamic scan of a running application and adjust its testing accordingly.

Robust Application Security with Strobes
Strobes employs comprehensive techniques to secure applications at their core, ranging from white box and black box security reviews to vulnerability testing. It provides:
- Proactive risk identification
- Enhanced security
- Protection against sophisticated attacks like OWASP top 10
- Complete compliance.
With a team of seasoned experts and advanced pen-testing methodologies, Strobes provides actionable insights to improve your security posture by multifold. With our state-of-the-art PTaaS(Pentesting-as-a-service), you can confidently unveil vulnerabilities, assess risks, and fortify your applications against cyber threats effectively – Request Pentest